Create an extendable HealthBar class with Phaser 3
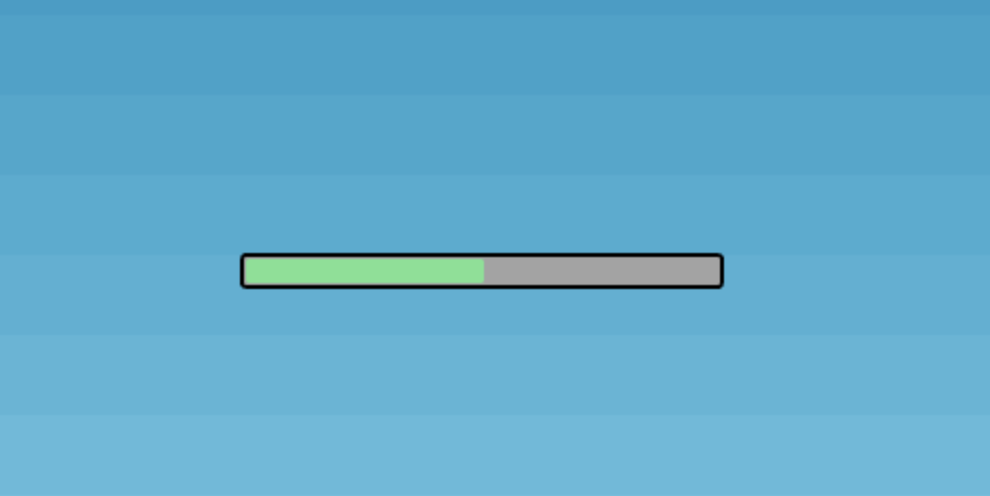
Welcome back to new tutorial everyone, in this post I will create an extendable Healthbar class that you can use for all of your character. Like enemy, player, boss etc. I have used this same version of healthbar class for implementing gotchi, enemy, turkey, gmls health in Aavegotchi Arena
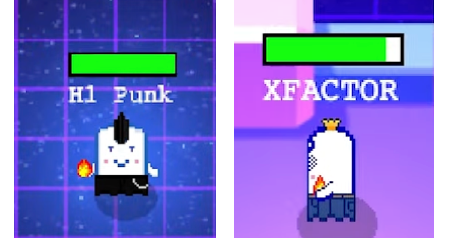
The HealthBar class
class HealthBar extends Phaser.GameObjects.Graphics {
constructor(scene, x, y, max = 1000) {
super(scene);
this.bar = this; // the Graphics object
this.x = x; // X coordinate of the healthbar
this.y = y; // Y coordinate of the healthbar
this.value = max; // current healthbar value (initialize with max value)
this.percentage = 238 / max; // get the percentage
this.maxHP = max; // set max healthbar
this.draw(); // draw the healthbar
this.setDepth(1); // set depth so it is always on top
scene.add.existing(this); // add to the scene
}
draw() {
this.bar.clear(); // clear the graphics
this.percentage = 238 / this.maxHP;
// background
this.bar.fillStyle(0xa3a3a3); // grey
this.bar.fillRoundedRect(this.x, this.y, 240, 16, 2);
// border
this.bar.lineStyle(2, 0x000000, 1);
this.bar.strokeRoundedRect(this.x, this.y, 240, 16, 2);
// health color
this.bar.fillStyle(0x74e291); // green
const dx = Math.floor(this.percentage * this.value);
this.bar.fillRoundedRect(this.x + 2, this.y + 2, dx, 12, 2);
}
// apply damage and get current health value
getDamage(amount) {
this.value = amount;
if (this.value < 0) {
this.value = 0;
}
this.draw();
return this.value === 0;
}
// return current health value
getCurrentHealth() {
return this.value;
}
}
instantiate the class from create method
function create() {
// instantiate healthbar object with spawn position and max health
const healthBar = new HealthBar(this, 100, 100, 1000);
// apply damage for testing
healthBar.getDamage(500);
}